If you want to automate some of your manual integration testing I can highly recommend WatiN. It is a great library for doing browser automation. The new version allows you to automate Internet Explorer and Firefox through a common interface.
example:
[Fact] public void SearchForCodingInstinctOnGoogle() { using (IBrowser ie = BrowserFactory.Create(BrowserType.InternetExplorer)) { ie.GoTo("http://www.google.com"); ie.TextField(Find.ByName("q")).Value = "Coding Instinct"; ie.Button(Find.ByName("btnG")).Click(); Assert.True(ie.ContainsText("Coding Instinct")); } }
I am using xUnit.NET in the above example (Fact = Test). xUnit.NET has been mentioned in a lot of blogs lately and I think for good reasons. It comes bundled with a very nice set of extensions that are very useful, especially for integration testing.
ExcelData:One of the extensions that comes with xUnit is an attribute that lets you fetch test data from an excel file, xUnit will then execute your test method for each row in the excel file. Here is an interesting usage scenario:
[Theory, ExcelData(@"Resources\PortalUrls.xls", "select * from PortalUrls")] public void UrlShowsExptectedContent(string url, string contentText, bool requireLogin) { InitBrowser(url); if (requireLogin) { LoginAction.WithAS(); } Assert.True(Browser.Text.Contains(contentText), string.Format("\"{0}\" was not found", contentText)); }
My current customer is using a portal application to host a number of separate applications (that use a common SSO). With this simple test I was able to check all portal URLs and with a very simple validation check that the applications (and the login) was up and running.
The excel file:
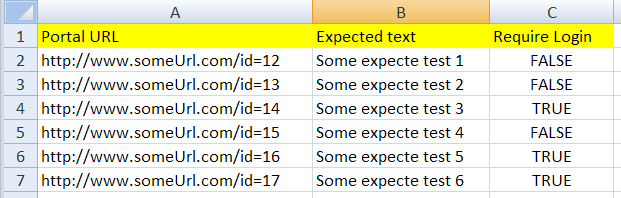
In the excel file you must use the function "Name a Range". This function can be found by selecting the table, including the header and right clicking. It is also found under the "Formulas" tab (Define Name, Name Manager).
The test above is of course a very simple test, It only checks that the portal is configured correctly and that all applications are up and running, but it is a good start. Now I can go into each application/feature and write automated tests for each of the manual test cases.
UI test fragility:When writing browser tests you need to do a lot of html element selections so the tests become very fragile to UI changes, this is especially true for WebForm applications where element names and ids are constantly changed for minor UI changes.
To combat this problem I tend to place most of the element selections in static helper classes. I also use a RegEx to identify elements. The reason for using a RegEx is to ignore a large part of the id prefix that the WebForm html renderer so gladly adds. example:
public class LoginAction : ActionBase { public static void WithAS() { Browser.TextField(new Regex(".*txtUserName")).Value = "xxxx"; Browser.TextField(new Regex(".*txtPassword")).Value = "xxxx"; Browser.Button(new Regex(".*btnLogin")).Click(); } }
There are many more useful xUnit extension attributes, for example: InlineData, PropertyData and SqlServerData. For more information on WatiN and xUnit.NET here are some links:
- WatiN homepage: watin.sourceforge.net
- xUnit.NET homepage: www.codeplex.com/xunit/
- Introdution to xUnit
- Introduction to XUnit.net Extensions