Your starting a new web project and you want to use sass/less for css and require.js for javascript dependency management. Maybe you want to use jslint/jshint to verify your javascript syntax and csslint to verify your css. To make this a smooth developing experience you want to run a background file watcher that runs tasks whenever you change a file.
So when you modify a javascript file, all js files are verified for syntax, require js optimizer is executed to traverse your dependency tree (and spits out combined files). Maybe javascript tests are executed on the fly as well. Same thing when you modify a sass file you want the generated css to be created behind the scenes automatically.
I have used psake (Powershell build script framework) for almost all my build scripts lately. I am not a big fan of Powershell, at least the Powershell syntax. But psake makes writing build scripts a lot easier than msbuild ever has.
I wrote a little powershell utility function that sets up file watchers that when triggered executes a psake task.
Example:
task Watch { WatchFiles "$scripts_dir" "*.js" "JsCheck" WatchFiles "$touch_test_proj_dir\JavaScript" "*.js" "JsCheck" WatchFiles "$styles_dir" "*.scss" "Sass" }
Whenever a js file is modified in the scripts folder (or subfolders) execute psake task JsCheck (this task will run jshint, require js optimizer, and javascript tests).
Here is the gist for the WatchFiles function:
The only trouble I had was dealing if duplicate events, the .NET file watcher class is a little buggy, generating duplicate file changed events which required a little hack to filter them out.
There are many file watchers for node / grunt but I found them a little buggy and CPU intensive. And by using powershell and a regular .NET file watcher does not block the console, you can still use the Powershell console that is running the file watchers.
Growl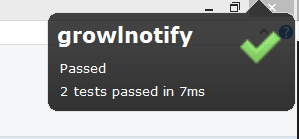
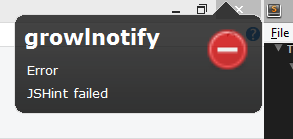
Another thing that is very nice to have when you run background tasks is notification when things go bad or good. This is where Growl for Windows is handy. If a powershell psake task throws an exception I can call growlnotify like this:
function growl($title, $message, $icon) { if (Test-Path "C:\Program Files (x86)\Growl for Windows") { $iconUri = ([system.uri] "$base_dir\build\images\$icon.png").AbsoluteUri Write-Host $iconUri & growlnotify /t:$title /i:"$iconUri" "$message" } }
That way you instantly know when you have missed a comma, broke the code style guidelines, wrote the wrong require js dependency path etc.